Signal Entity¶
API Documentation: [cpp] [python] [.NET] [LabVIEW]
SignalClass. Interface to digital pins configured to produce square wave signals. This class is designed to allow for square waves at various frequencies and duty cycles. Control is defined by specifying the wave period as (T3Time) and the active portion of the cycle as (T2Time). See the entity overview section of the reference for more detail regarding the timing.
Signal entity to Digital entity mapping varies from device to device. Please refer to the datasheet.
Timing¶
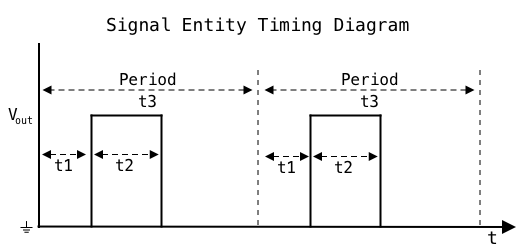
Set/Get enable¶
signal [ index ] . getEnable <= (unsigned char) enable
signal [ index ] . setEnable => (unsigned char) enable
Enables the Signal Entity for a given index.
Set/Get T3 Time¶
signal [ index ] . getT3Time <= (unsigned int) t3_nsec
signal [ index ] . setT3Time => (unsigned int) t3_nsec
The T3 time defines the period of the waveform in nano seconds.
Set/Get T2 Time¶
signal [ index ] . getT2Time <= (unsigned int) t2_nsec
signal [ index ] . setT2Time => (unsigned int) t2_nsec
The T2 time defines the high period of the waveform in nano seconds.
Set/Get invert¶
signal [ index ] . getInvert <= (unsigned char) invert
signal [ index ] . setInvert => (unsigned char) invert
Inverts the meaning of the T2 time. When inverted the T2 time will represent the time in nano seconds that the waveform is low.
Code Examples¶
C++¶
//Setup 10Hz Signal Output with 50% Duty Cycle
err = stem.digital[0].setConfiguration(digitalConfigurationSignalOutput);
err = stem.signal[0].setT2Time(50000000);
err = stem.signal[0].setT3Time(100000000);
err = stem.signal[0].setEnable(1);
//Setup Signal as input and calculate the duty cycle.
err = stem.digital[4].setConfiguration(digitalConfigurationSignalInput);
err = stem.signal[4].getT2Time(&t2Time);
err = stem.signal[4].getT3Time(&t3Time);
double dutyCycle = ((double)t2Time / t3Time) * 100;
Python¶
#Setup 10Hz Signal Output with 50% Duty Cycle
err = stem.digital[0].setConfiguration(digitalConfigurationSignalOutput);
err = stem.signal[0].setT2Time(50000000);
err = stem.signal[0].setT3Time(100000000);
err = stem.signal[0].setEnable(1);
#Setup Signal as input and calculate the duty cycle.
err = stem.digital[4].setConfiguration(digitalConfigurationSignalInput);
t2Time = stem.signal[4].getT2Time();
t3Time = stem.signal[4].getT3Time();
dutyCycle = (t2Time.value / t3Time.value) * 100;