aEther¶
Overview¶
What is it?¶
aEther is a software feature that encapsulates the BrainStem software object and provides synchronization across multiple threads, processes and computers by way of a client-server model.
How does aEther help me utilize Acroname hardware?¶
Simplified Synchronization: Tired of working with Mutexes? Simply create and connect to an object for each thread and we will handle the rest.
Improved Debugging: Want to know what’s going on with Acroname hardware while your application is running or paused on a break point? No problem, just open up HubTool and monitor the devices state.
Remote Access: Is remote desktop too sluggish or overkill? With the proper network configuration you can access your devices from the other side of the world.
Communication Models¶
Models define a specific pattern or behavior. These behaviors have different attributes that might be more applicable for specific situations. The BrainStem protocol supports multiple communications models. By default the Client-Server model (aEther) is used because it is the most user friendly and adaptable.
Client-Server Model (aEther)¶
The Client-Server model works by routing all traffic through the operating systems socket layer. When the first connection is made a “server” and “client” pair will be created. All subsequent connections will be “clients” only. Note that the server is owned by the first user to connect to the device. Deletion of that object will result in ALL clients being disconnected. The server encapsulates the device by way of the direct connection model (discussed below). By doing this the server can manage simultaneous access of many clients.
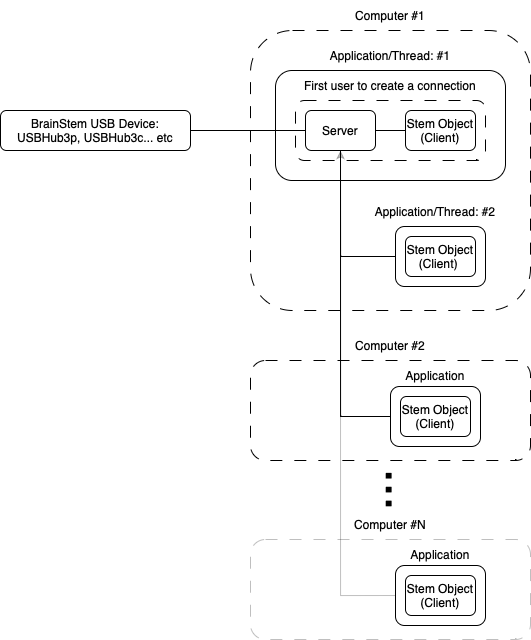
Configuring the device for external exposure¶
By default all sockets are restricted to the localhost. In other words, by default these sockets do NOT have access to the outside world and therefore should not trigger any firewall warnings. In the event you do want the device to be accessible remotely it simply needs to be enabled. Generally speaking this only exposes it to your local network; further exposure to the internet will depend on configurations outside of BrainStem.
#include "BrainStem2/BrainStem-all.h"
USBHub3c stem;
Acroname::BrainStem::aEtherConfig config;
stem.getConfig(&config);
config.localOnly = false;
//NOTE: This must be done before connection
stem.setConfig(config);
stem.discoverAndConnect(USB)
import brainstem
stem = brainstem.stem.USBHub3c()
config = stem.getConfig()
config.localOnly = False
#NOTE: This must be done before connection
stem.setConfig(config)
stem.discoverAndConnect(brainstem.link.Spec.USB)
Configuring the device for a specific network adapter¶
Sometimes it is necessary to define the network interface you would like to use. If your system has many network adapters (including virtual ones) you might need to programmatically configure this setting. Typically, this is only common for the following cases.
Hosting a device for external access (localOnly = False) - By default the first interface will be used. Typically, this is the default interface. If the default is not the desired interface you will need to configure it as shown below.
Discovery - By default discovery will search the localhost only; however, if you provide a valid network interface it will search that instead. This is particularly important if using stem.discoverAndConnect() for a remote device that is not directly connected to the computer. The network interface of the module must be set before calling a connect function as shown below.
Note that you can programmatically request the available interfaces on your machine through “aDiscovery_GetIPv4Interfaces”
#include "BrainStem2/BrainStem-all.h"
USBHub3c stem;
Acroname::BrainStem::aEtherConfig config;
stem.getConfig(&config);
config.networkInterface = 0xA00A8C0; //Example: 192.168.0.10 (network byte order)
config.localOnly = false;
//NOTE: This must be done before connection
stem.setConfig(config);
stem.discoverAndConnect(USB)
import brainstem
stem = brainstem.stem.USBHub3c()
config = stem.getConfig()
config.networkInterface = 0xA00A8C0 #Example: 192.168.0.10 (network byte order)
config.localOnly = False
#NOTE: This must be done before connection
stem.setConfig(config)
stem.discoverAndConnect(brainstem.link.Spec.USB)
Disabling auto fallback¶
In order to make a more pleasant user experience, the “discoverAndConnect” process will automatically check other transport types for devices if a device is unavailable with the given transport type. For instance, we want to create 2x BrainStem software objects that connect to the same USB module (ie USBHub3p etc). The first caller should use the transport type “USB” while the second should use “AETHER”. When fallback is enabled, this can be ignored and the underlying software will automatically determine the appropriate transport for you. If you use the “USB” transport and it is determined that someone is already connected to this device it will automatically try the “AETHER” transport next. Depending on your situation you might not want this behavior. If so, it can be disabled through the NetworkConfig.
#include "BrainStem2/BrainStem-all.h"
USBHub3c stem;
Acroname::BrainStem::aEtherConfig config;
stem.getConfig(&config);
config.fallback = false;
//NOTE: This must be done before connection
stem.setConfig(config);
stem.discoverAndConnect(USB)
import brainstem
stem = brainstem.stem.USBHub3c()
config = stem.getConfig()
config.fallback = False
#NOTE: This must be done before connection
stem.setConfig(config)
stem.discoverAndConnect(brainstem.link.Spec.USB)
Disabling the Client-Server model¶
There might be specific situations in which you want to disable the client-server model and revert to the previous direct connection model paradigm. This is very simply done through the aEtherConfig structure.
#include "BrainStem2/BrainStem-all.h"
USBHub3c stem;
Acroname::BrainStem::aEtherConfig config;
stem.getConfig(&config);
config.enabled = false;
//NOTE: This must be done before connection
stem.setConfig(config);
stem.discoverAndConnect(USB)
import brainstem
stem = brainstem.stem.USBHub3c()
config = stem.getConfig()
config.enabled = False
#NOTE: This must be done before connection
stem.setConfig(config)
stem.discoverAndConnect(brainstem.link.Spec.USB)
Direct Connection Model¶
The direct connect model is Acroname’s original model and is the core of the server in the client-server model. In this model the BrainStem software object owns the device communications path and is generally thread safe. When using this model the device is NOT accessible in any other processes nor is it accessible by another computer. This model is slightly faster, but more restrictive.
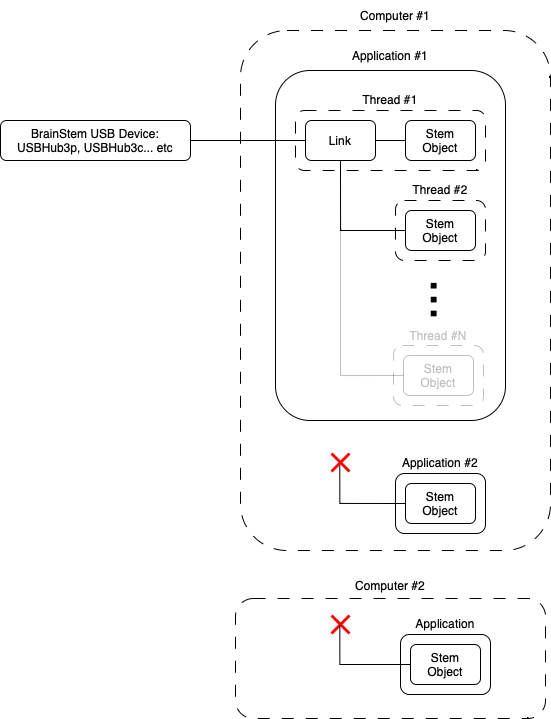
To select this configuration it must be done through the aEtherConfig structure.
#include "BrainStem2/BrainStem-all.h"
USBHub3c stem;
Acroname::BrainStem::aEtherConfig config;
stem.getConfig(&config);
config.enabled = false;
//NOTE: This must be done before connection
stem.setConfig(config);
stem.discoverAndConnect(USB)
import brainstem
stem = brainstem.stem.USBHub3c()
config = stem.getConfig()
config.enabled = False
#NOTE: This must be done before connection
stem.setConfig(config)
stem.discoverAndConnect(brainstem.link.Spec.USB)