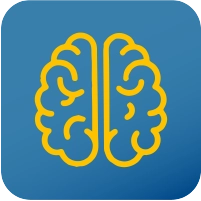
Automate your USB devices with APIs
Unlock Efficiency and Precision: Automating USB Devices Made Easy
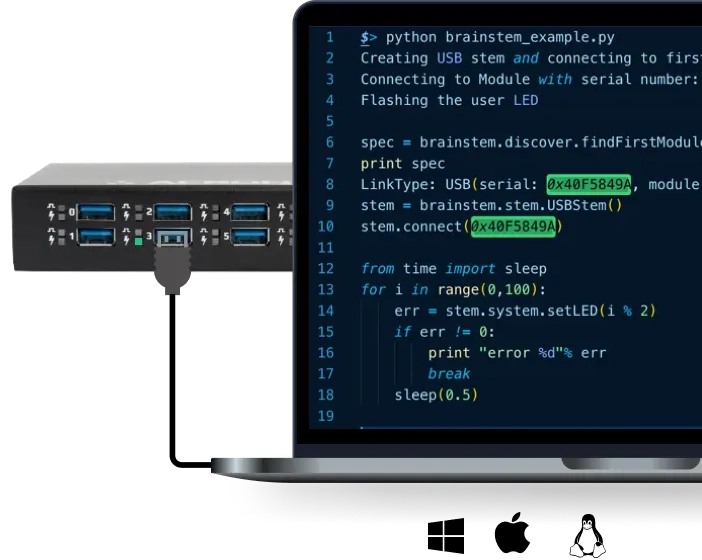
Pick a language, get a serial number
Copy code
import brainstem
stem = brainstem.stem.USBHub3c()
result = stem.discoverAndConnect(brainstem.link.Spec.USB)
if result == (brainstem.Result.NO_ERROR):
result = stem.system.getSerialNumber()
print("Connected to USBHub3c with serial number: 0x%08X" % result.value)
else:
print ('Could not find a module.\n')
stem.disconnect()
Copy code
#include
#include "BrainStem2/BrainStem-all.h"
int main(int argc, const char * argv[]) {
std::cout << "Creating a USBHubc module" << std::endl;
aUSBHub3c hub;
aErr err = aErrNone;
uint32_t serial_number = 0;
err = hub.discoverAndConnect(USB);
if (err != aErrNone) {
std::cout << "Error "<< err <<" encountered connecting to BrainStem module" << std::endl;
return 1;
} else {
std::cout << "Connected to BrainStem module." << std::endl;
stem.system.getSerialNumber(&serial_number);
std::cout << "Serial Number: " << serial_number << std::endl;
}
stem.disconnect();
}
Copy code
int main(int argc, const char * argv[]) {
linkSpec* spec = NULL;
aLinkRef stem = 0;
aErr err = aErrNone;
spec = aDiscovery_FindFirstModule(USB, LOCALHOST_IP_ADDRESS);
if ((spec != NULL) &&
(spec->t.usb.usb_id != 0))
{
stem = aLink_CreateUSB(spec->t.usb.usb_id);
if (0 == stem) {
printf("Error creating link.\n");
err = aErrConnection;
goto cleanup;
}
}
else{
printf("No BrainStem module was discovered.\n");
err = aErrNotFound;
goto cleanup;
}
//Wait for link to transition to running state.
uint8_t count = 0;
while ((aLink_GetStatus(stem) != RUNNING) &&
(count < 30))
{
count++;
aTime_MSSleep(10);
}
if ((count >= 30) &&
(aLink_GetStatus(stem) != RUNNING))
{
printf("BrainStem connection failed!\n");
err = aErrNotReady;
goto cleanup;
}
printf("Connected to BrainStem module %08X\n", spec->t.usb.usb_id);
cleanup:
if(spec) { aLinkSpec_Destroy(&spec); }
if(stem) { aLink_Destroy(&stem); }
return err;
}
Copy code
curl http://127.0.0.1:9005/api/v1/devices
# OR
curl http://127.0.0.1:9005/api/v1/acronameDevices
# Endpoint responses will contain an object with a connected Acroname device's serial number
Copy code
using System;
using System.Threading;
using Acroname.BrainStem2CLI;
namespace BrainStem2CLI_USBHub3c
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Creating USBHub3c module");
USBHub3c stem = new USBHub3c();
UInt32 serial_number = 0;
aErr err = stem.module.discoverAndConnect(m_linkType.USB, 0);
if (err != aErr.aErrNone)
{
Console.WriteLine("Error connecting to device: {0}", err);
Console.WriteLine("Press enter to exit");
Console.ReadLine();
System.Environment.Exit(1);
}
else
{
Console.WriteLine("Successfully connected to BrainStem module\n");
stem.system.getSerialNumber(ref serial_number);
Console.WriteLine("Serial Number: {0}", serial_number);
}
stem.module.disconnect();
}
}
}
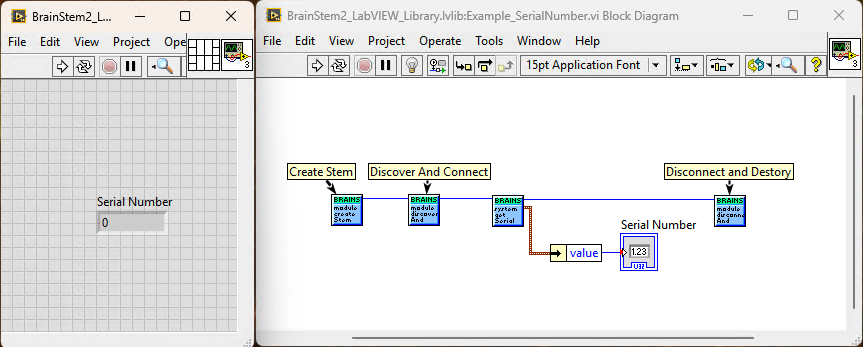