USB¶
API Documentation: [cpp] [python] [.NET] [LabVIEW]
The USB Entity provides the software control interface for USB related features. This entity is supported by BrainStem products which have programmatically controlled USB features.
Alt. Mode Configuration (Redriver Only)¶
The redriver model USB-C-Switch provides an intermediary receiver and amplifier on the HS and SS data lines. Various alt-modes such as DisplayPort require different directional uses of the SS data lines. As such, it is required to define the alt-mode and direction of the connection. These modes are responsible for setting the direction of the SS data lines and related SBU lines.
stem.usb.getAltModeConfig(0, configuration)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setAltModeConfig(0, configuration)
[cpp]
[python]
[NET]
[LabVIEW]
where configuration is an integer value defined below. Details of the pin mapping and data direction are also depicted below.
Index |
Alt Mode Configuration |
---|---|
0 |
USB 3.1 Disabled |
1 |
USB 3.1 Enabled |
2 |
4 Lane DisplayPort Host on Common Port |
3 |
4 Lane DisplayPort Host on Mux Port |
4 |
2 Lane DisplayPort with USB 3.1 – Host on Common Port |
5 |
2 Lane DisplayPort with USB 3.1 – Host on Mux Port |
6 |
2 Lane DisplayPort Host on Common Port with USB 3.1 Inverted |
7 |
2 Lane DisplayPort Host on Mux Port with USB 3.1 Inverted |
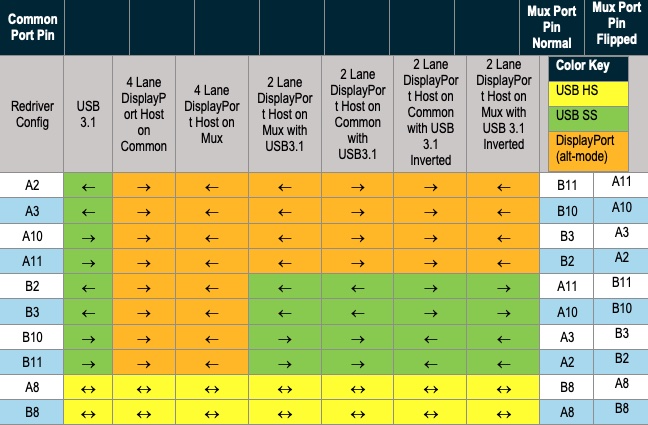
Cable Flip¶
The USB-C-Switch can simulate a cable flip by electrically switching the CC/VCONN and SBU lines between side-A and side-B of the USB-C female sockets. USB data lines are also swapped accordingly. This flip can be done with:
stem.usb.getCableFlip(setting)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setCableFlip(setting)
[cpp]
[python]
[NET]
[LabVIEW]
where the parameter (setting) is an interger value of 0 or 1, where 0 is normal and 1 is full cable flip.
Individual functional groups of the USB connection can be flipped using the portMode option.
CC Manipulation¶
The automatic orientation detection and connection functionalite is interfaced with:
stem.usb.setConnectMode(0, mode)
[cpp]
[python]
[NET]
[LabVIEW]
where (mode) is a Boolean value of 0 or 1.
Manipulating the CC lines is done by calling:
stem.usb.setCC1Enable(0, enabled)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setCC2Enable(0, enabled)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.getCC1Enable(0, enabled)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.getCC2Enable(0, enable)
[cpp]
[python]
[NET]
[LabVIEW]
where (enable) is a Boolean vale of 0 or 1.
CC line current and voltage can be measured with:
stem.usb.getCC1Voltage(0, µV)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setCC2Voltage(0, µV)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.getCC1Current(0, µA)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.getCC2Current(0, µA)
[cpp]
[python]
[NET]
[LabVIEW]
where positive current is power transfer from the common port to the mux port.
Channel Control¶
The usb entity provides a mechanism to control and monitor all USB functionality on the common port. Individual parts of the USB connection can be manipulated through the usb entity. For example, enable/disable USB data and Vbus lines, measure current and voltage on Vbus, VCONN, and CC. The USB-C-Switch has one usb entity class. It uses the mux entity to select one of the 4 mux channels to which to connect the enabled USB signals.
The usb entity splits the USB connection into tree going from most generic to most specific with usb entity options at each level. Higher levels of the tree can be used to cause simultaneous changes on the lower levels. The tree structure is port(Vbus, data(HS, SS), USB-C(CC1, CC2, SBU)).
The usb.setPortEnable/Disable entity option allows for manipulating all parts of the USB connection (HS data, SS data, both CC and SBU lines, and Vbus lines) simultaneously.
stem.usb.setPortEnable(channel)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setPortDisable(channel)
[cpp]
[python]
[NET]
[LabVIEW]
Where channel is always 0 for the USB-C-Switch. Further examples of the usb entity will always show the channel option as 0.
Manipulating USB data lines (HS and SS) simultaneously is done by calling:
stem.usb.setDataEnable(0)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setDataDisable(0)
[cpp]
[python]
[NET]
[LabVIEW]
Manipulating HS or SS data lines is done by calling:
stem.usb.setHiSpeedDataEnable(0
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setHiSpeedDataDisable(0)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setSuperSpeedDataEnable(0)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setSuperSpeedDataDisable(0)
[cpp]
[python]
[NET]
[LabVIEW]
Manipulating Vbus lines are done by calling:
Port Manipulation¶
Vbus voltage and current through the switch’s Vbus lines can be measured with:
stem.usb.getPortVoltage(0, µV)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.getPortCurrent(0, µA)
[cpp]
[python]
[NET]
[LabVIEW]
where positive current is power transfer from the common port to the mux port.
Port Mode¶
The portMode option provides a bitmapped setting for granular control of the individual connections. The portMode option is the desired mode of the port. The companion option, portState, is used to provide the current state of the port.
stem.usb.getPortMode(0, mode)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.setPortMode(0, mode)
[cpp]
[python]
[NET]
[LabVIEW]
where (mode) is a 32-bit word, defined below.
Bit |
Port Mode Bit Map |
---|---|
0 |
Reserved |
1 |
Reserved |
2 |
Keep Alive Charging Enable |
3 |
Reserved |
4 |
HS Side A Data enable |
5 |
HS Side B Data enable |
6 |
Vbus enable |
7 |
SS Lane 1 Data enable |
8 |
SS Lane 2 Data enable |
9:10 |
Reserved |
11 |
Auto Connect enable |
12 |
CC1 enable |
13 |
CC2 enable |
14 |
SBU enable |
15 |
CC Flip enable |
16 |
Super-Speed Flip enable |
17 |
SBU Flip enable |
18 |
Hi-Speed Flip enable |
19 |
CC1 Current Injection enable |
20 |
CC2 Current Injection enable |
21:31 |
Reserved |
Port Operational State¶
The portState option provide an interface to the state of the common port and internals of the USB-C-Switch system.
stem.usb.getPortState(0, state)
[cpp]
[python]
[NET]
[LabVIEW]
where (state) is a 32-bit word, defined below.
Bit |
Port State Bit Map |
---|---|
0 |
Vbus enable |
1 |
HS Side A Data enable |
2 |
HS Side B Data enable |
3 |
SBU enable |
4 |
SS Lane 1 Data enable |
5 |
SS Lane 2 Data enable |
6 |
CC1 enable |
7 |
CC2 enable |
8:9 |
Reserved |
10:11 |
Reserved |
12:13 |
Reserved |
14 |
CC Flip enable |
15 |
Super-Speed Flip enable |
16 |
SBU Flip enable |
17 |
Reserved |
19:18 |
Daughter-Card status |
22:20 |
Reserved |
23 |
Connection Established |
24:25 |
Reserved |
26 |
CC1 Current Injection |
27 |
CC2 Current Injection |
28 |
CC1 Pulse detect |
29 |
CC2 Pulse detect |
30 |
CC1 Logic state |
31 |
CC2 Logic state |
SBU Manipulation¶
stem.usb.setSBUEnable(0, enabled)
[cpp]
[python]
[NET]
[LabVIEW]
stem.usb.getSBUEnable(0, enabled)
[cpp]
[python]
[NET]
[LabVIEW]
where (enable) is a Boolean vale of 0 or 1.