Getting Power Data Objects from Acroname API
If you've used HubTool, you've probably seen this helpful screen:
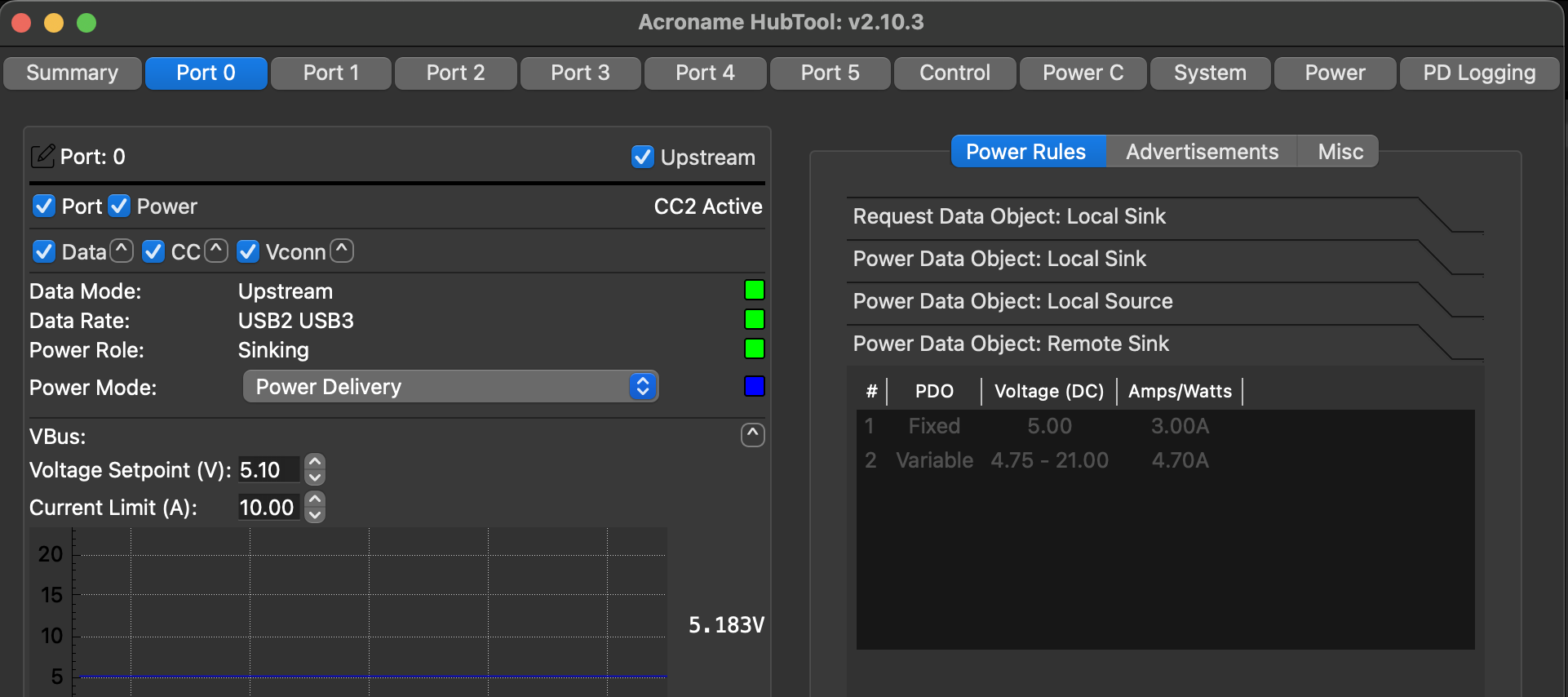
And perhaps thought: "I'd really like to use those values (5.00 VDC, 3A) in my python script!"
And possibly tried Acroname's API call to Get A Particular Power Data Object (PDO). e.g.
>>> c_hub.pd[4].getPowerDataObject(1, 1, 1).value
654413974
Which seems like it ought to return "Fixed, 5.00 volts, 1.5 amperes", but instead returns some integer "654,413,974". The USB 3.2 Power Delivery Standard defines PDO's encoding as a sequence of 32-bits. And the Acroname API returns that sequence in its integer representation. Some decoding work must be done by the user to make this useful.
Decoding Integers into helpful things
I'm using "USB_PD_R3_2 V1.1 2024-10.pdf", from the The USB 3.2 Power Delivery Standard. Particularly, sections 6.4.1.1, and 6.4.1.2.1.
Beware: The encoding of PDOs is ambiguous without the context of "sourcing" or "sinking" category. "Sourcing", what kind of power can be provided, is detailed in section 6.4.1.2. "Sinking", what kind of power can be received, is detailed in section 6.4.1.3. Avoid the wrong section when decoding PDOs.
654,413,974 has a binary representation as
0010 0111 0000 0001 1001 0000 1001 0110 (separated into nibbles for convenience)
With bit[31] on the very left, and bit[0] on the very right.
Table 6.7 in the specification lays out the first part of the parsing grammar. In this case, we parse left to right, or rather, starting at the big-end (bit[31]).
Bit(s) | Description | ||
---|---|---|---|
Value | Parameter | ||
B31…30 | 00b | Fixed Supply (Vmin = Vmax) | |
01b | Battery | ||
10b | Variable Supply (non-Battery) | ||
11b | Augmented Power Data Object (APDO) | ||
B29…0 | Specific Power Capabilities are described by the PDOs in the following sections. |
PDO := (0b00 fixed_supply_pdo_message)
| (0b01 battery_pdo_message)
| (0b10 variable_supply_pdo_message)
| (0b11 augmented_pdo)
The first two bits [31...30] serve as a discriminator. Bits [29...0] serve as one of four possible messages. Bits[29...0] are equivalent to a a union
in C, or a std::variant
in C++. Looking at our integer 654,413,974:
0010 0111 0000 0001 1001 0000 1001 0110 (separated into nibbles for convenience)
We see at our discriminator is 0b00: The following bits are a Fixed Supply message, which is detailed in Section 6.4.1.2.1, Table 6.9.
Bit(s) | Field | Description |
---|---|---|
B31…30 | Fixed Supply | 00b - Fixed Supply PDO |
B29 | Dual-Role Power | Set to ‘1’ for Dual-Role Power device. |
B28 | USB Suspend Supported | Set to ‘1’ if USB suspend is supported. |
B27 | Unconstrained Power | Set to ‘1’ if unconstrained power is available. |
B26 | USB Communications Capable | Set to ‘1’ if capable of USB Communications capable |
B25 | Dual-Role Data | Set to ‘1’ for a Dual-Role Data device. |
B24 | Unchunked Extended Messages Supported | Set to ‘1’ if Unchunked Extended Messages are supported. |
B23 | EPR Capable | Set to ‘1’ if EPR Capable. |
B22 | Reserved | Reserved – Shall be set to zero. |
B21…20 | Peak Current | Peak Current value. |
B19…10 | Voltage | Voltage in 50mV units |
B9…0 | Maximum Current | Maximum current in 10mA units |
Ah. There's "Voltage" in bits[19...10]. Let's do some quick python math to see the voltage of this PDO:
0010 0111 0000 0001 1001 0000 1001 0110 (separated into nibbles for convenience)
I've italicized the bits[19...10] that are interesting for voltage: 0b0001100100.
We take this quanta value, and multiply it by 50mV units:
>>> 0b0001100100 * 0.050
5.0
5.0 volts. Which is exactly what we'd expect for the first PDO object.
To do the bit-field extraction programmatically:
>>> x = 654413974 # Start with the integer representation of the PDO
>>> x = (x >> 10) # Right-shift '10' so that bits [19...10] become bits [9...0]
>>> x = x & ((1 << 10) - 1) # bitwise-AND with a 10-bit-wide mask, so that only bits [9...0] remain.
And then:
>>> voltage = x * 0.050
Or in a one-liner for voltage:
voltage = 0.050 * (c_hub.pd[4].getPowerDataObject(1, 1, 1).value >> 10) & ((1 << 10) - 1)
Same deal with current, in bits[9...0]:
>>> x = 654413974 # Start with the integer representation of the PDO
>>> x = (x >> 0) # Right-shift '0' so that bits [9...0] become bits [9...0]
>>> x = x & ((1 << 10) - 1) # bitwise-AND with a 10-bit-wide mask, so that only bits [9...0] remain.
>>> current = 0.010 * x # Ten milliamps per quanta.
1.5
Or 1.5 amperes maximum current.
--
And that's how you take the returns of "getPowerDataObject" and turn them into useful values -- reference the USB 3.2 Power Delivery spec, extract the bitfields, and multiply them by the Specification's units.
Add New Comment