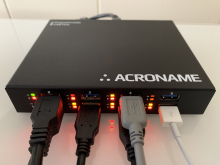
Expand Hub Functionality with BrainStem API
Acroname's hubs are a robust hardware solution to many common usb related tasks: remotely simulating a manual plug-cycle, charging (or not charging) batteries on connected devices, and switching control between two host computers.
Accompanying this robust hardware is an equally robust, mature, freely available suite of software in the form of a 'HubTool GUI' and our BrainStem API. Available in multiple languages and for multiple operating systems, these tools make programming and interacting with our devices easy and intuitive. Our BrainStem API in particular provides easy access to scripting opportunities that can expand the functionality of your Acroname hub.
A common support question we see is 'If I'm running a a script, how can I tell if a device is attached on a particular port? Can I tell what kind of device (USB2 or USB3) is attached?" The answer is an enthusiastic, "Yes - both!"
Here's some example code in python2/3 for our 8-port USBHub3p that will connect to an attached USBHub3p, cycle the hub's 8 downstream ports, and return information on each port in the form of a bit-packed integer that can be bit-masked and shifted to get USB device attachment information. Note: This code snippet requires that you have downloaded our BrainStem Development Kit and installed the included python BrainStem wheel.
# This file is a modified version of an example from of the BrainStem development package.
# For easy access to error constants
from brainstem.result import Result
USB2_BIT_MASK = 0x800
USB3_BIT_MASK = 0x1000
DEVICE_BIT_MASK = 0x800000
print ('\nCreating USBHub3+ hub object and connecting to first module found')
stem = brainstem.stem.USBHub3p()
# Locate and connect to the first USBHub3+ you find on USB
result = stem.discoverAndConnect(brainstem.link.Spec.USB)
# Locate and connect to a specific module (replace with your Serial Number (hex) if multiple Acroname devices attached)
# example:
# result = stem.discoverAndConnect(brainstem.link.Spec.USB, 0x66F4859B)
# Check error
if result == (Result.NO_ERROR):
result = stem.system.getSerialNumber()
print ("Connected to USBHub3+ with serial number: 0x%08X" % result.value)
print ('Getting state for all ports:\n')
for port in range(0, 8):
port_state_raw = stem.usb.getPortState(port)
port_state = port_state_raw.value
print(f"port {port} state is: {hex(port_state)}")
device_attached = bool((port_state & DEVICE_BIT_MASK) >> 23)
usb2_device_attached = bool((port_state & USB2_BIT_MASK) >> 11)
usb3_device_attached = bool((port_state & USB3_BIT_MASK)>> 12)
print("Device attached on port %d: %s" % (port, device_attached))
print("USB2 device attached on port %d: %s" % (port, usb2_device_attached))
print("USB3 device attached on port %d: %s" % (port, usb3_device_attached))
else:
print ('Could not find a module.\n')
# Disconnect from device
stem.disconnect()
This code is a modified version of our USBHub3p python example code, also found inside the BrainStem Dev Kit, which is always a good place to start when writing your own scripts. Our python API documentation contains these API calls as well as many more. The bit-packed values can be found in the USBHub3p data sheet, page 11. A similar chunk of code will work for our 4-port USBHub2x4 with modifications such as bit-masking and bit-shifting values, the hub connection code - see the USBHub2x4 data sheet and python example in our Brainstem Dev Kit to get started.
Your Acroname hub has lots of additional functionality beyond what's visible on the surface - stroll the API documentation when you have time, and please reach out to Acroname support if you have any questions about your particular application.
Add New Comment